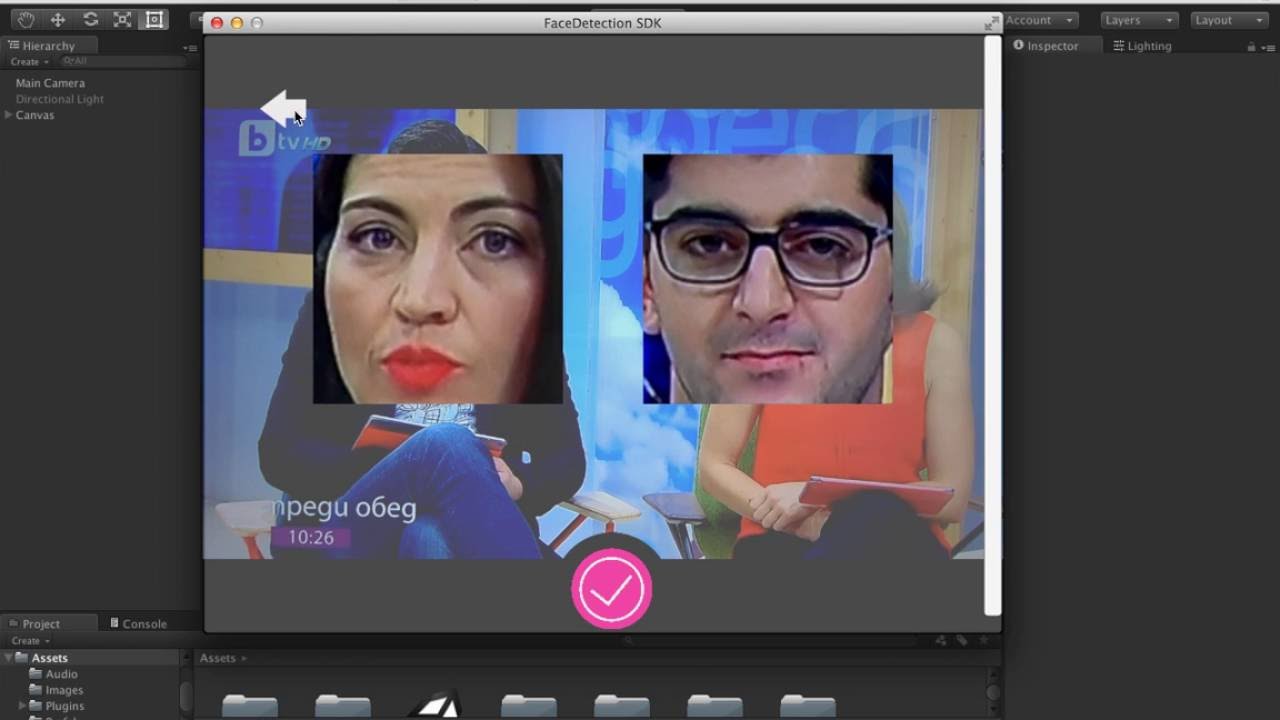
Face Detection Lib Face Tracking Sdk Quantum Vision
In this step you'll create the basic skeleton of an app that you'll fill in later by adding the sign in code.
Buenas Bruno, Have you tried to perform face recognition using the KinectSdk? I've tried a simple algorithm that calculates the euclidean.
Create the new App
Open Android Studio. When you launch Android Studio, you'll see an option to ‘Start a new Android Studio project'. Select this. You'll see the ‘New Project' dialog. Enter the details for your app.
Press Next, and you'll get a screen asking to ‘Target Android Devices'. Accept the default here, and press Next to the next screen.
You'll see ‘Add an activity to Mobile' dialog. Ensure that you select ‘Blank Activity' here.
Click ‘Next' and you'll be asked to customize the Activity. Just accept the defaults, and press ‘Finish'.
Configure Build.gradle
In this step you'll ensure that your app can use Google Play services, in which the Mobile Vision APIs reside. To do this, you'll first update your build.gradle file.
In Android Studio, open the Gradle Scripts node, and select build.gradle (Module App) as shown:
This will open your build.gradle file, at the bottom of which will be code like this:
Add a dependency for play services like this:
If you are asked to perform a gradle sync, do so. Otherwise, find the Gradle Sync button on the toolbar and press it to trigger a sync. It looks like this:
Update Google Play Services
Google Play services is frequently updated and this codelab assumes you have a recent version. To get the latest version, in Android Studio click Tools > Android > SDK Manager:
Then find the entry for Google Play Services and make sure you have version 26 or higher:
Build a Basic UI
Now that your app is fully configured, it's time to build a UI that lets the user detect a face in an image, and then overlay that face with a bounding box.
In Android Studio, select the ‘res' folder, and open it's ‘layout' subfolder. In here you'll see ‘activity_main.xml
'.
Double click to open it in the editor, and be sure to select the ‘Text' tab at the bottom of the editor to get the XML text view of your Layout. Android Studio Should look something like this:
You can see that your layout contains a single <TextView>
node. Delete this and replace with:
This layout gives you a a loading and then processing an image, which will appear in the ImageView.
Edit your AndroidManifest.xml
You should edit your AndroidManifest.xml file at this point with the following line:
This ensures that the libraries are available for face detection.
Adding face detection to your app
Typically you would take pictures with the device's camera, or maybe process the camera preview. That takes some coding, and in later steps you'll see a sample that does this. To keep things simple, for this lab, you're just going to process an image that is already present in your app.
Here's the image:
Image - 'Woman and a chiwawa dog' by Peter van der Sluijs, available on the CC 3.0 license, downloaded from here
Name it test1.jpg, and add it to the res/drawable directory on your file system. You'll see that Android Studio adds it to the drawable directory. It also makes the file accessible as a resource, with the following ID: R.drawable.test1
With the image in place, you can now begin coding your application.